18-348 Lab #6
Spring 2016
NOTE: Lab 6 consists of two components (Lab 6 Part A and Lab 6 Part
B).
Relevant lectures:
- Part A: Lecture 10. Serial Ports
- Part B: Lecture 11. Debug and Test
Links to all files referenced in the lab and prelab can be found in the
Files section at the end of this document.
Navigation
Pre-Lab
Lab
Support Materials
Goal:
- To learn the basics of serial communication.
Discussion:
Refer to Chapter 13 of the
MCU9S12
Datasheet for details of the Serial Communication Interface. Refer to
the lecture notes for details on serial communication.
Procedure:
Part 1:
Study Sections 13.3 and 13.4 of the
MCU9S12
Datasheet.
For each table below, fill in the values from the corresponding list (Hint
there are the same number of values as locations in the table. Each value
should be used only once).
Table 1:
Register
|
Value for this bit=0
|
Value for this bit=1
|
SCICR1 bit 7
|
|
|
SCICR1 bit 6
|
|
|
SCICR1 bit 5
|
|
|
SCICR1 bit 4
|
|
|
SCICR1 bit 3
|
|
|
SCICR1 bit 2
|
|
|
SCICR1 bit 1
|
|
|
SCICR1 bit 0
|
|
|
Values for table 1:
(copy each phrase below into exactly one box above)
- 8 bit data format
- 9 bit data format
- Count idle bits after start bit
- Count idle bits after stop bit
- Disable loop operation (Normal operation)
- Disable SCI in wait mode
- Enable loop operation
- Enable SCI in wait mode
- Even parity
- Odd Parity
- Parity disabled
- Parity enabled
- Receiver connected externally to transmitter
- Receiver internally connected to transmitter output
- Wake on address mark
- Wake on idle line
Table 2:
Register
|
Value for this bit=0
|
Value for this bit=1
|
SCICR2 bit 7
|
|
|
SCICR2 bit 6
|
|
|
SCICR2 bit 5
|
|
|
SCICR2 bit 4
|
|
|
SCICR2 bit 3
|
|
|
SCICR2 bit 2
|
|
|
SCICR2 bit 1
|
|
|
SCICR2 bit 0
|
|
|
Values for Table 2:
- IDLE interrupt disabled
- IDLE interrupt enabled
- No break characters
- No receiver wakeup (normal operation)
- RDRF interrupt disabled
- RDRF interrupt enabled
- Receiver disabled
- Receiver enabled
- Receiver wakeup and block receiver interrupts
- TC interrupt disabled
- TC interrupt enabled
- TDRE interrupt disabled
- TDRE interrupt enabled
- Transmit break characters
- Transmitter disabled
- Transmitter enabled
Table 3:
Register
|
Value=0
|
Value=1
|
SCISR1 bit 7
|
|
|
SCISR1 bit 6
|
|
|
SCISR1 bit 5
|
|
|
SCISR1 bit 4
|
|
|
SCISR1 bit 3
|
|
|
SCISR1 bit 2
|
|
|
SCISR1 bit 1
|
|
|
SCISR1 bit 0
|
|
|
Values for Table 3:
- Data not available in SCI data register
- Framing error detected
- No byte in transmit shift register
- No framing error detected
- No noise detected
- No overrun
- No parity error detected
- Noise detected
- Overrun has occurred
- Parity error detected
- Received data in SCI data register
- Receiver idle
- Receiver not idle
- Transmission complete
- Transmission in progress
- Transmit register empty
Part 2:
Review section 13.4.2. of the
MCU9S12
Datasheet on baud rate generation. Note that the values in table
13-10 are incorrect for our purposes because they assume a module clock rate of
25 MHz.
For this lab we will assume that the module clock rate is 8 MHz. The
following calculations should be performed assuming the clock speed is 8
MHz.
Using the formula given, fill in the SCIBR[12:0] values required for each
of the listed baud rates below. Compute the actual baud rate (using the
formula and the integer value you chose for SCIBR. Compute the % error
using the formula:
100 * (Actual Baud Rate - Nominal Baud Rate) / Nominal Baud Rate
Table 4:
Nominal Baud Rate (bits / sec)
|
SCIBR value (decimal representation)
|
Actual Baud Rate (bits / sec)
|
Error (%)
|
300
|
|
|
|
600
|
|
|
|
1200
|
|
|
|
2400
|
|
|
|
4800
|
|
|
|
9600
|
|
|
|
14400
|
|
|
|
19200
|
|
|
|
38400
|
|
|
|
56000
|
|
|
|
115200
|
|
|
|
Bonus: Part 3 (Optional)
Implement a trigonometric sine function using fixed point math (4 bits
integer; 12 bits fraction). This function is used for drawing arcs, generating
acoustic waveforms, and other purposes in embedded systems. For the prelab,
design a suitable algorithm. The design representation can be any reasonable
mixture of mathematical formulas and a flowchart. The design:
- Shall compute correct outputs for all possible fixed point inputs.
- Shall assume inputs are in fixed point radians, and outputs are fixed point
values in the same 4 bit/12 bit format as inputs.
- Can use any reasonable method of range reduction of inputs, including
iterated subtraction instead of division.
- Can use any reasonable computation method.
- Shall produce results with at least 11 bits accuracy after the radix point.
- Shall cite the source of any external information used (e.g., the source of
a formula). It is OK to find the math for this somewhere (but tell us the
source!). It is not OK to copy or substantially copy code.
Remember this is an individual assignment. Your test plans should be done independently. For the prelab only put your name in the spreadsheet.
Goal:
To write a testing plan
Discussion:
For this part, you will plan a white-box testing on a statechart
implementation of a coin receiver for a vending machine. The state chart
is shown below:
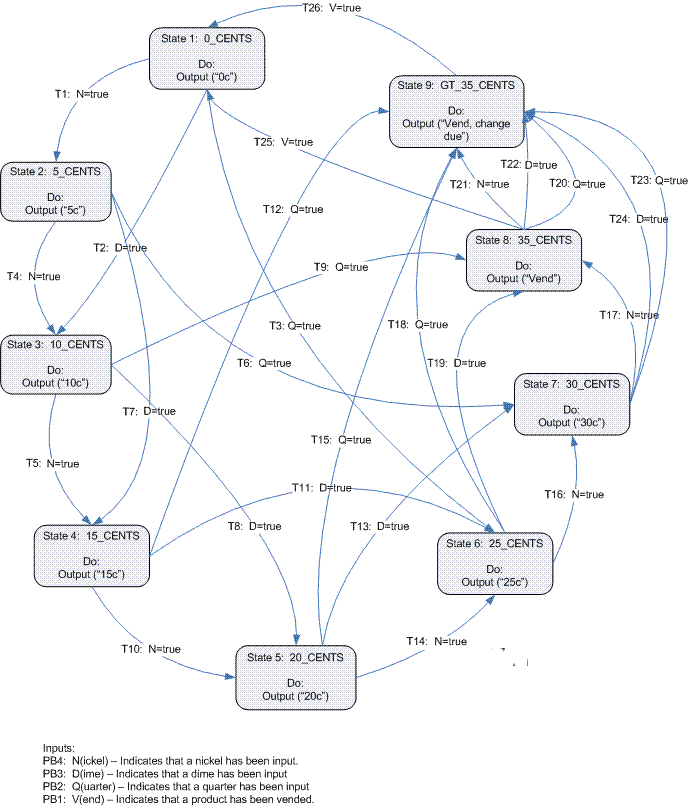
The tests you will implement are white-box tests in the sense that you will
test the transitions in the state chart shown above. For the purposes of
testing, you may assume that no more than one input is asserted at one time
(e.g. N and D should not be pressed simultaneously).
An example test sequence for this implementation is given in the table
below. The state in parentheses is the state that the controller should
be in after the input. If your test reveals that the actual state is
different than the expected one, you should note the actual state in the
description column of T2.3 (e.g. "Transition Tx goes to state 4 instead of
state 5.").
|
Pass/Fail
|
Initial State
|
Input 1 (State after
input) |
Step 2 (State after input)
|
Step 3 (State after input)
|
Step 4 (State after input)
|
Test 2.1
|
PASS
|
1
|
N (2)
|
N (3)
|
N (4)
|
N (5)
|
Table 1: Test Sequences
The above test sequence tests arcs T1, T4, T5, and T10. The coverage of
the test is recorded in the table below by placing an X in the appropriate
columns. This documents traceability of tests to arcs, answering the questions
"which arcs does a particular test exercise?" and "for a given
arc, which tests exercise that arc?"
Table 2: Traceability (Tests to Arcs)
Tables 1 & 2 together form a White Box Test Plan of a type commonly used
in embedded industry.
Procedure:
- Download the the testplan_template.xls
file (use the WB_* sheets). Rename it to testplan_<andrewid>.xls.
- Write additional tests for the statechart implementation. You tests
must meet the following requirements:
- There shall be no fewer than 5 tests.
- Every transition in the statechart shall be tested by at least one test.
- All tests shall start in state #1 (the reset state).
- Update the traceability table for the tests that you write. Use the format
of Table 2 above.
- Create a results table based on the expected results of the test using the
format of Table 1 above. But, don't actually do the tests yet. This means that
the "pass/fail" column will be blank, but all other columns will be
filled in.
- Bonus (optional): Create a black box testing plan for the requirements in the Lab Part B.3: fill out Tables 4 & 5 from the Lab (black box testing plans) leaving the results blank. This is just a test plan, you'll perform the tests during the lab and finish the table.
Prelab Hand-in Checklist: (90 + 14 pts)
All non-code and non-test plan submissions shall be in a single PDF
document.
Part A:
- (10 points) Complete Table 1 from part 1.
- (10 points) Complete Table 2 from part 1.
- (10 points) Complete Table 3 from part 1.
- (20 points) Complete Table 4 from part 2.
- (Bonus 10 points) Submit your design for the fixed point math sine function
in Part 3. The design representation can be any reasonable mixture of
mathematical formulas and a flowchart.
Part B:
- (40 points -- 20 points for each table) Your completed test plan and
results tables (in format of tables 1-2 above) with all test plan information.
Please submit this as a PDF file that prints with at least 10 point font
(as-printed size) and fits each row on no more than one sheet of paper across.
Landscape format is OK to give a little more row width. Do NOT submit in
.xls format. We are using PDF files so we do not need to support multiple
versions of MS Office.
- (Bonus: 4 points) Hand in completed Tables 4 & 5 (black box tests from
the Lab description below) except, leave results blank (so this is only a
test plan, not a completed report of testing). This is individual work, but
you are encouraged to merge these tests with your lab partner's
after you hand in your prelab to accomplish more thorough
testing.
Refer to the LAB FAQ for more information on lab
handin procedures and file type requirements. You MUST follow these
procedures or we will not accept your submissions.
Goal:
- To learn the basics of serial communication
Discussion:
For this lab, you will establish serial communication with a PC. We
have written a simple program which reads a query string from the serial port,
looks for the query in a string file, and returns a response string over the
serial port. The program uses the following protocol:
- Each character of the message (excluding the null terminator) is
transmitted.
- Then a Cyclic Redundancy Code (CRC) of the message is transmitted.
- Then a NULL byte is sent to signal the end of transmission.
Your program must be able to follow this protocol when it sends or receives
data from the PC.
In addition to the PC software, you will use an LCD library to display
output on the onboard LCD. lcd_lib-1_2.zip contains information about
how to use it.
Also, you will use a clock modification library to increase the APS12C128
module bus clock from 2 MHz to 8 MHz. Add the files in
modclock.zip to your project.
Procedure:
Part 1: PC serial program.
- Determine the address of the serial port (in Windows XP) by doing the
following:
- Open the control panel.
- Open "System Properties"
- Select the Hardware tab.
- Click the Device Manager button
- Expand the Ports item in the tree.
- You should see a list of com ports, e.g. "Communications Device
(COM1)." If you are using a USB to serial adapter, you may see the
brand-name of the adapter.
- Download the SerialComm program. The
zip file contains a sample string table file, the executable, and several
supporting DLLs. The DLLs must be in the same directory as the
executable.. You can obtain the command line syntax of the program by
running it with no arguments.
Part 2: Hardware and Software
- Wire the board according to the comments in the skeleton file. Be
sure to check the jumper settings on the default jumper settings page.
- Download the lab 6 skeleton file,
modclock.zip, and
lcd_lib.zip.
- Start a new C project. Rename the skeleton to lab_6_gXX.c and replace
the main file with it. Add the modclock.h, modclock.c, lcd_lib.h and
lcd_lib.c files.
- Implement the serial communications by filling in the TODO sections of the
program. The program must meet the following requirements:
- After reset, the program shall display the current groupString, the
computed CRC, and the current baud rate index.
- When PB1 is pressed, the program shall send a 2-byte string containing the
group name over the serial connection (according to the communications protocol
described above)
- After transmitting the query string, the program shall receive a response
from the PC, decode the response, and check the message integrity using the
CRC.
- The program shall display the response and the correct CRC or an error if
the CRC check fails.
- The program may terminate after 1 transmission cycle (i.e. need to be reset
before another query/response).
- Switches SW1_1 to SW1_4 should be used to set serial communication at the
different Baud rates
Part 3: Run the system
- Test your program by connecting the DB9 port on the Project Board with
the serial port on the PC.
- Run the SerialComm program using the demo.txt
and initiate a communication.
- Write out the byte-by-byte values of the query and response
communications. The default groupString has been done below as an
example.
- Query: 0x45, 0x58, 0xA6, 0x00
- Response: 0x53, 0x61, 0x6d, 0x70, 0x6c, 0x65, 0xc8, 0x00
Part 4: Communication Speeds
Try the communication at each baud rate, starting at 300 baud and working
up. Record the fastest baud rate that you can successfully communicate at
and the slowest baud rate where communication fails (usually with a frame error
on the PC). In other words, record the range of baud rates at which communication works, along with boundary values. Note that you will have to demo these two speeds to the TA,
so if you are doing the part of the lab on a computer other than one in the
lab, you must be able to bring the computer with you to lab for the demo.
Include the fastest and slowest values in your write up.
Bonus - Part 5: (Optional)
Write a subroutine that computes sin(x) for fixed point input values X in
radians (4 bits integer; 12 bits fraction; two's complement inputs; same format
for outputs). See the corresponding prelab bonus question for more information.
Debugging hint: you can generate a large number of automated tests by using the
identity sin(X)**2 + cos(X)**2=1 as an oracle to test correct execution. It is
also OK to check output values against outputs from a floating point C library.
Look at the corresponding writup question before you write this code.
Part A - Demo Checklist: (48 + 20 points)
For the demo, the TA may ask you to use a different string file. The
query string will always be your lab number (e.g. "1A" in ASCII
characters). Response strings will never be longer than 8 characters.
- (20 points) Demo successful communication between the module and the
computer at 2400 baud.
- (20 points) Demo successful communication between the module and the
computer at 9600 baud.
- (8 points) Demo your findings for the fastest baud rate with
successful communication and the slowest baud rate where communication fails
(if all work, make sure you show your TA).
- (Bonus - 20 points) Optional. The TA will randomly pick four angles X in
radians within the range of inputs. Compute sin(X) and the TA will record it
for later checking. Use your choice of input and output methods, including
debugger memory access.
Goal:
To write test plans and use various testing techniques to test an existing
codebase.
Discussion:
In this lab, you will not write any code. Instead, you will write test
plans for code which we will supply to you. The supplied code is the
absolute assembly file (with debugging symbols removed). The steps below
describe the procedure for loading the code.
Here is how to load .abs files using the Hiwave debugger:
- Connect the USB cable to the PC and the project board. Make sure the
board is powered.
- Load C:\Program Files\Freescale\CodeWarrior for S12(X) V5.0\prog\hiwave.exe
(The exact path on your machine may vary).
- Choose MultilinkCyclonePro >
Communication... from the menu. Choose USB-PEMICRO_SHARED.
Click OK.
- Choose MultilinkCyclonePro > Set
Derivative... from the menu. Choose MC9S12C128 from the drop box
if it is not already chosen. Click OK.
- Choose MultilinkCyclonePro > Load...
from the menu. If the Load...
option is not available (grayed out), you may need to choose the
Reset option first.
- In the Load dialog box, select the .abs file you wish to load. Check
the Options for "Automatically erase and
program into FLASH and EEPROM", "
Verify memory image after loading code
" and "Run after successful
load".
- Click OK for the "Warning: No Debug Info" message.
- At this point, the .abs file should be executing on your module.
Probably you will want to use a spreadsheet program to create these tables,
just as they do in industry. But, please turn in Acrobat files as usual, and
make sure that the table text is large enough to read (at least 10 point as
printed on the page -- not squished down to tiny-type font size!) without
requiring us to tape pages together due to wide rows.
Note: If you don't see MultilinkCyclonePro on the menu, to make it appear,
do the following
1. Component -> Set Connection
2. Processor HC12, Connection P&E Multilink/Cyclone Pro
3. OK
Now you should see MultilinkCyclonePro as one of the choices on the menu.
Procedure:
To get full coverage (and full points), you must find at least two bugs in
Part 2 and one bug in Part 3.
Part 1:
Wire the board according to the following list:
- Connect PB1 to AD00
- Connect PB2 to AD01
- Connect PB3 to AD02
- Connect PB3 to AD03
- Connect the onboard LEDs to PT0:PT7
- Make sure the LCD_EN jumpers MOSI, MISO, and SCK are connected on the
project board.
- The contrast jumper should be set to FIX
- The SS jumper should be set to SS*.
Part 2 (White Box Testing)
After you have handed in your pre-labs, compare your test
plans from the pre-lab and merge them to create a better test plan. (You can
just use one of your two test-plans as-is if they both look OK to you. We don't
care which of the two you pick if they are both good plans.)
A bug identified by your test should be tracked in a bug list as in table
2.3 below. The columns in this table are as follows: The bug # is a
unique number assigned to each bug for tracking purposes. Test # should
be the test that identified the bug. Transitions should be a list of any
transitions that are affected by the bug. Description is a detailed
description of the problem (e.g. "Transition Txx causes a transition to
state X instead of state Y.").
Bug #
|
Test #
|
Transition(s)
|
Description
|
|
|
|
|
Table 3: Buglist
- Load the file whitebox.abs using the procedure
described in the discussion section.
- Download the the testplan_template.xls
file (use the WB_* sheets for part 2). Rename it to testplan_gXX.xls.
- Merge your pre-lab white box tests for the statechart implementation, and
add any additional tests that might be needed if you discover gaps in your
pre-lab test plans. You tests must meet the following requirements:
- There shall be no fewer than 5 tests.
- Every transition in the statechart shall be tested by at least one test.
- All tests shall start in state #1 (the reset state).
- Update the traceability table for the tests that you write based on merging
your two pre-labs. Use the format of Table 2 above.
- Carry out the tests and record the results in the pass/fail column of a
results table based on merging your two pre-labs. Use the format of Table 1
above.
- Record any bugs you find in the bug list sheet. Use the format of Table 3
above.
Part 3: (Black Box Testing)
In this part you will do black box testing on an implementation of the
wiper controller similar to the one you wrote for Lab 3. You will develop
a test plan that includes an acceptance test to cover every requirement. The
wiring from part 1 should work for part 3 as well. PB1 is used to
initiate the OFF state, PB2 for INTERMITTENT, PB3 for SLOW, and PB4 for FAST.
The requirements for this implementation are listed below:
- R1. The controller shall have the operating modes OFF, INTERMITTENT,
SLOW, FAST.
- R2. When turned OFF, the wiper blades shall return to the rest
position.
- R3. Each mode may only be entered from its adjacent mode according to
the sequence in R1.
- R4. INTERMITTENT wiper speed shall be such that the wipers complete a
cycle every 2 seconds (+/- 0.1 seconds), with 2 seconds (+/- 0.1 seconds)at the
rest position between each cycle.
- R5. SLOW wiper speed shall be such that the wipers complete a cycle
every 2 seconds (+/- 0.1 seconds) with no delay between cycles.
- R6. FAST wiper speed shall be such that the wipers complete a cycle
every 1 second (+/- 0.1 seconds) with no delay between cycles.
- R7. A delay in any required operating mode transition of up to one
full wiper cycle is acceptable.
A framework for testing each requirement is shown in the table below:
|
Pass/Fail
|
Test Description
|
Result |
Test 3.1
|
PASS
|
Verify the timing of FAST
MODE using a stopwatch to measure average cycle time over 10 cycles.
|
Wiper cycle average time
is 0.98 seconds.
|
Table 4: Test result table with example entry.
The example test shown above tests requirement R6. This is reflected
by the X in the traceability table below.
|
R1
|
R2
|
R3
|
R4
|
R5
|
R6
|
R7
|
Test 3.1
|
|
|
|
|
|
X
|
|
Table 5: Traceability (Tests to Arcs)
A bug identified by your test should be tracked in a bug list as in table 6
below. The columns in this table are as follows: The bug # is a
unique number assigned to each bug for tracking purposes (for example: B1, B2,
B3, ...). Test # should be the test that identified the bug.
Requirement(s) should be a list of any requirements that are affected by the
bug. Description is a detailed description of the problem (e.g.
"Transition Txx causes a transition to state X instead of state Y.").
Bug #
|
Test #
|
Requirements(s)
|
Description
|
|
|
|
|
Table 6: Buglist table.
- Load the file
blackbox.abs using the procedure described in
the discussion section.
- Use the file testplan_gXX.xls to record your test plan and results (use the
BB_* sheets for part 3).
- Write additional acceptance tests for the requirements. You tests
must meet the following requirements:
- There shall be no fewer than 5 tests.
- Every requirement shall be tested by at least one test.
- Fill out a traceability table for the tests that you write. (Use format of
Table 5.)
- Carry out the tests and record the results in ta test result table.
Determine if the test passes or fails and indicate that in the Pass/Fail
column. (Use format of Table 4.)
- Record any bugs you find in a bug list table. (Use format of Table 6.)
Part B - Questions:
- For white-box testing (part 2), how much coverage of the statechart
transitions did your tests achieve?
- For black-box testing (part 3), how much coverage of the requirements did
your tests achieve?
- Are these tests sufficient to ensure that there are no bugs? Why or
why not? (Limit your answer to 100 words).
- Hypothesize at least one bug that might not be detected by a test plan that
covers 100% of the transitions in the statechart from Part 2. (Limit your
answer to 100 words).
Part B - Demo Checklist: (50 points)
- (20 points) Carry out the tests that failed (i.e. identified bugs)
from Part 2.
- (20 points) Carry out the tests that failed (i.e. identified
requirements that had not been met) from Part 3.
- (10 points) Carry out two tests chosen by the TA at random from your
test plan.
Lab Hand-in Checklist: (127 + 25 pts)
Part A:
- (5 points) List any problems you encountered in the lab and pre-lab, and
suggestions for future improvement of this lab. If none, then state so to get
these points.
- (20 pts) Submit your completed C implementation (as lab_6_gXX.c).
Your code must conform to the course style sheet to receive full credit.
- (25 points) Record the byte-by-byte query and response (using your own
group name) from part 3.
- (10 points) List the fastest baud rate with successful communication and
the slowest baud rate where communication fails from part 4.
- (Bonus: 25 points) Optional. Give the code listing for your sin(X) routine
as executed on the microcontroller. Port the subroutine to a desktop computer
and write an automated test that checks all 64K possible input values for
accuracy. Hand in files that we can use gcc (or gxx) to compile to run your
tests (we would strongly prefer exactly one .h and exactly one .c or .cpp file
to make it easier for us to build and run your program).
Part B:
Test plans may be included in your write-up PDF or as an additional PDF
file. Test plans must have at least 10 point font (as-printed size) and fit
each row on no more than one sheet of paper across. Landscape format is OK to
give a little more row width. NOTE: Do NOT submit in .xls format. We are
using PDF files so we do not need to support multiple versions of MS
Office.
- (5 points) List any problems you encountered in the lab and pre-lab, and
suggestions for future improvement of this lab. If none, then state so to get
these points.
- (10 points -- 5 points for each table) Hand in your revised white box test
plan, with results filled in (Tables 1-2).
- (40 points -- 10 points for each table) Hand in the rest of your completed
test plan and results tables (Tables 3-6) with all test plan information and
test results.
- (12 points -- 3 points for each question) Answer the questions for
part 4.
Refer to the LAB FAQ for more information on lab
handin procedures and file type requirements. You MUST follow these
procedures or we will not accept your submissions.
Part A:
- There are reports of lab computers having problems if both the USB cable
and a serial port device are plugged in at the same time. If you have problems
downloading firmware try unplugging the serial cable (leaving the USB cable
attached) when you download.
Part B:
If you are using Excel, the following options generally help with meeting
the format requirements:
- You want cells to "wrap text" and not "shrink to fit".
So if you have a lot of text the box just grows down instead of text slopping
over the cell box. Command sequence for Excel 2003: (Format | Cells | Alignment
| Wrap text)
- Probably you want to choose landscape paper orientation (not portrait)
Command sequence for Excel 2003: (File | Page setup | Page | Landscape)
- You want to size columns so each row fits on a single sheet across. This is
easier if you display page breaks. Command sequence for Excel 2003: (View |
Page break preview); adjust column sizes; (View | normal)
- To make it easier to generate a single Acrobat file, you might wish to add
a tab for answering questions.
- To print everything in a single Acrobat file, select "entire
workbook" on the print menu.
- Caution: Excel might resize pages a little depending on your printer, so be
careful. We've tried to set up the example spreadsheet for you, but it might be
slightly off due to the vagaries of Excel.
FILES for this lab:
Part A:
Part B:
Relevant reading:
Also, see the course materials
repository page.
Change notes for 2016:
- 3/21/16 - Added clarification to baud rate range. -- Milda
- 2/25/16 - Updated demo.txt in SerialComm zip (now SerialCommv4.zip) -- Milda
- 2/19/16 - Removed extraneous transitions from traceability xls -- Milda
- 2/5/16 - Added navigation -- Milda